Git Cheat Sheet
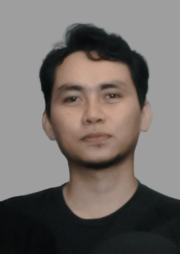
Panduan singkat menggunakan git untuk melakukan version control di segala projek
JagoTekno.com - Git merupakan aplikasi version control yang hampir digunakan oleh semua developer.
Git dapat membantu kita memonitor source kode yang sedang dibuat. Kita bisa melakukan roollback terhadap perubahan yang sudah pernah dilakukan kemudian melakukan sejumlah modifikasi.
Di git kita juga bisa melakukan kolaborasi dengan orang lain untuk mengerjakan satu atau lebih projek secara bersama-sama tanpa saling terganggu.
Git dicintai oleh semua developer karena semua kemudahan yang dimilikinya sangat memudahkan pengembangan aplikasi.
Mari kita mulai
Daftar Isi
Create a Repository
- Create a new local repository
git init [project-name]
- Clone an existing repository
git clone [url]
- Clone a specific branch
git clone -b [branch] [url]
- Clone a specific branch and create a new directory
git clone -b [branch] [url] [new-directory]
Configuration
- Set the name that will be attached to your commits and tags
git config --global user.name "[name]"
- Set the email address that will be attached to your commits and tags
git config --global user.email "[email address]"
- Edit the global configuration file in a text editor
git config --global --edit
- Set a default branch name other than master
git config --global init.defaultBranch [branch-name]
- Enable some colorization of Git output
git config --global color.ui auto
- Set Git to use the credential memory cache
git config --global credential.helper cache
- Set Git to use credential memory cache for 1 hour
git config --global credential.helper 'cache --timeout=3600'
Make a change
- Show modified files in working directory
git status
- Stages the file, ready for commit to HEAD
git add [file]
- Stage all changed files, ready for commit to HEAD
git add .
- Stages all modified and deleted files, ready for commit to HEAD
git add -A
- Commit all local changes in tracked files
git commit -a
- Commit previously staged changes
git commit -m "[descriptive message]"
- Commit all local changes in tracked files
git commit -a -m "[descriptive message]"
- Unstages file, but preserve its contents
git reset [file]
- Discard all local changes in your working directory
git reset --hard
- Diff of what is changed but not staged
git diff
- Diff of what is staged but not yet commited
git diff --staged
- Diff of what is changed between two branches
git diff [first-branch]...[second-branch]
- Apply any commits of current branch a HEAD of specified one
git rebase [branch]
- Discard all history and changes back to the specified commit
git reset --hard [commit]
Bekerja dengan Branch
- List all existing branches
git branch -a
- List all existing and remote branches
git branch -r
- List all branches, local and remote, in long format
git branch -a -v
- List all branches, local and remote, in long format with commit info
git branch -a -v --abbrev-commit
- Switch HEAD branch
git checkout [branch-name]
- Create a new branch based on your current HEAD
git branch [branch]
- Delete the specified branch
git branch -d [branch-name]
- Delete the specified branch, even if it has not been merged
git branch -D [branch-name]
- Rename a branch and its reflog
git branch -m [old-branch] [new-branch]
- Switch to the branch
git checkout [branch-name]
- Switch to the branch and create it if it doesn’t exist
git checkout -b [branch]
- Create a new tracking branch based on a remote branch
git checkout --track [remote-branch]
- Switch to the branch, and discard all changes in working directory
git checkout -f [branch-name]
- Merge the specified branch’s history into the current one
git merge [branch]
- Tag the current commit with a version number
git tag [tag]
Remote Repositories
- Add a new remote
git remote add [shortname] [url]
- Show the names of the remote repositories you’ve added
git remote
- List all currently configured remote repositories
git remote -v
- Show information about a remote repository
git remote show [remote]
- Remove a remote repository
git remote rm [remote]
- Change a remote’s URL
git remote set-url --push [remote] [newUrl]
Synchronize
- Fetch all branches from remote repository
git fetch --all
- Fetch all branches from remote repository and prune
git fetch --all --prune
- Merge a remote branch into your current branch to bring it up to date
git merge [remote]/[branch]
- Transfers commits, files, and refs from one remote to another
git push [remote] [branch]
- Fetch and merge any commits from the tracking remote branch
git pull
- Merge just one specific commit from another branch into your current branch
git cherry-pick [commit]
- Download all history from the repository
git fetch --unshallow
Tracking path Changes
- Show all commit logs with indication of any paths that moved
git log --stat -M
- Change an existing file path and stage the move
git mv [existing-path] [new-path]
- Delete a file from the working tree and stage the deletion
git rm [file]
- Remove a file from the working tree and stage the removal
git rm --cached [file]
- Add a file as if it was deleted and then re-created
git rm --cached -r .
Temporary Commits
- Save modified and staged changes
git stash
- List stack-order of stashed file changes
git stash list
- Write working from top of stash stack
git stash pop
- Discard the changes from top of stash stack
git stash drop
Ignoring Files
Ignoring Files adalah fitur git untuk menandai file / direktory mana yang tidak perlu dimasukken ke git sehingga tidak perlu dilihat oleh orang lain.
- Create a .gitignore file
touch .gitignore
- Add a file to .gitignore
echo "file.txt" >> .gitignore
- Add a directory to .gitignore
echo "directory/" >> .gitignore
- Add a file extension to .gitignore
echo "*.extension" >> .gitignore
- Add a file pattern to .gitignore
echo "file*" >> .gitignore
- Add a file extension pattern to .gitignore
echo "*.ext*" >> .gitignore
- Add a exclude pattern to .gitignore
echo "!file.txt" >> .gitignore
- Add a exclude pattern to .gitignore
echo "!directory/" >> .gitignore
- Add a exclude pattern to .gitignore
echo "!*.extension" >> .gitignore
Git Tricks
Rename branch
- Rename your current branch
git branch -m new-name
- Rename any branch
git branch -m old-name new-name
- Push the new branch and delete the old one
git push origin :old-name new-name
- Reset the upstream branch for the new name
git push origin -u new-name
- Delete the old branch
git branch -d old-name
Commit amend
- Amend the most recent commit
git commit --amend
- Amend the most recent commit with a new message
git commit --amend -m "New commit message"
- Amend the most recent commit with the staged changes
git commit --amend --no-edit
- Amend the most recent commit with the staged changes and a new message
git commit --amend -m "New commit message" --no-edit
Log
- Show the commit history for the currently active branch
git log
- Show the commit history for the currently active branch, including all commits from all branches and remotes
git log --all --graph --decorate --oneline
- Show the commit history for the currently active branch, including all commits from all branches and remotes, and the diffs between each commit
git log --all --graph --decorate --oneline --patch
- Show out visual representation of the commit history
git log --graph --oneline --decorate --all
- Search the commit history for the given author
git log --author="[name]"
- Search the commit history for the given commit message
git log --grep="[message]"
- Search the commit history for the given file
git log -- [file]
- Search the commit history for the given file, including diffs
git log -p -- [file]
- Search the change by connected with the given commit
git log -S [string]
Revert
- Revert a commit by creating a new commit
git revert [commit]
- Revert a commit by creating a new commit, and edit the commit message
git revert -m 1 [commit]
Reset
- Reset the current HEAD to the specified state
git reset [commit]
- Reset the current HEAD to the specified state, and keep the changes in the working tree
git reset --keep [commit]
Git Aliases
- Create a new alias
git config --global alias.[alias] [command]
- Create a new alias with arguments
git config --global alias.[alias] "[command] [arguments]"
- Create a new alias with arguments and options
git config --global alias.[alias] "[command] [arguments] --[option]"
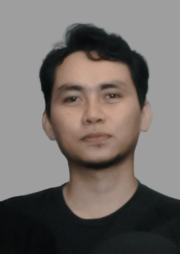
Rafi
- 15 year+ of Linux user.
- 5 years+ blogger and web developer.
Jika artikel yang dibuatnya ternyata bermanfaat, support dengan cara